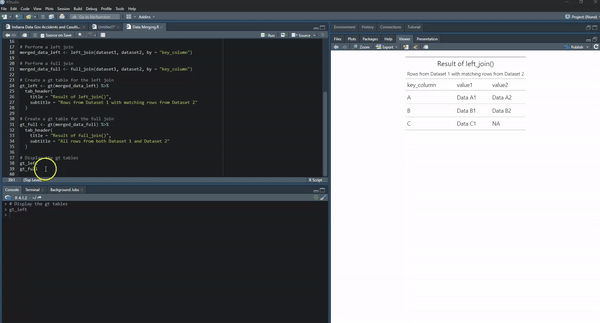
The Problem:
Manually merging datasets.
Errors creep in. Rows get lost.
It’s a slow, frustrating grind.
The Hack:
R fixes this.
Use left_join()
for smart merging.
It matches rows by a common key.
Example:
Here’s what you start with:
Dataset 1:
key_column | value1 |
---|---|
A | Data A1 |
B | Data B1 |
C | Data C1 |
Dataset 2:
key_column | value2 |
---|---|
A | Data A2 |
B | Data B2 |
D | Data D2 |
Run this code:
library(dplyr)
merged_data <- left_join(dataset1, dataset2, by = "key_column")
Result:
key_column | value1 | value2 |
---|---|---|
A | Data A1 | Data A2 |
B | Data B1 | Data B2 |
C | Data C1 | NA |
What happened to D2?
left_join()
only keeps keys from Dataset 1.
Key D
in Dataset 2 isn’t in Dataset 1, so it’s ignored.
To include D2
, use full_join()
instead:
merged_data <- full_join(dataset1, dataset2, by = "key_column")
Result with full_join():
key_column | value1 | value2 |
---|---|---|
A | Data A1 | Data A2 |
B | Data B1 | Data B2 |
C | Data C1 | NA |
D | NA | Data D2 |
Your Move:
Choose the right join for the job.
Use left_join()
for one-sided merges.
Use full_join()
to capture everything.